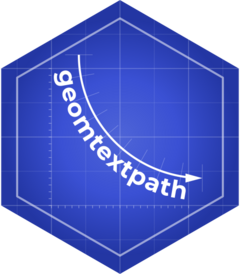
Produce labelled contour lines of 2D density in ggplot2
Source:R/geom_textdensity2d.R
geom_textdensity2d.Rd
Contour lines representing 2D density are available already in
ggplot2, but the native geom_density_2d
does not allow the lines to be labelled with the level of each contour.
geom_textdensity2d
adds this ability.
Usage
geom_textdensity2d(
mapping = NULL,
data = NULL,
stat = "density_2d",
position = "identity",
...,
contour_var = "density",
n = 100,
h = NULL,
adjust = c(1, 1),
lineend = "butt",
linejoin = "round",
linemitre = 10,
na.rm = FALSE,
show.legend = NA,
inherit.aes = TRUE
)
geom_labeldensity2d(
mapping = NULL,
data = NULL,
stat = "density_2d",
position = "identity",
na.rm = FALSE,
show.legend = NA,
inherit.aes = TRUE,
...,
contour_var = "density",
n = 100,
h = NULL,
adjust = c(1, 1),
lineend = "butt",
linejoin = "round",
linemitre = 10,
label.padding = unit(0.25, "lines"),
label.r = unit(0.15, "lines"),
arrow = NULL
)
Arguments
- mapping
Set of aesthetic mappings created by
aes()
oraes_()
. If specified andinherit.aes = TRUE
(the default), it is combined with the default mapping at the top level of the plot. You must supplymapping
if there is no plot mapping.- data
The data to be displayed in this layer. There are three options:
If
NULL
, the default, the data is inherited from the plot data as specified in the call toggplot()
.A
data.frame
, or other object, will override the plot data. All objects will be fortified to produce a data frame. Seefortify()
for which variables will be created.A
function
will be called with a single argument, the plot data. The return value must be adata.frame
, and will be used as the layer data. Afunction
can be created from aformula
(e.g.~ head(.x, 10)
).- stat
The statistical transformation to use on the data for this layer, as a string.
- position
Position adjustment, either as a string, or the result of a call to a position adjustment function.
- ...
Other arguments passed on to
layer
. These are often aesthetics, used to set an aesthetic to a fixed value, likecolour = "red"
orsize = 3
. These can also be the following text-path parameters:gap
A
logical(1)
which ifTRUE
, breaks the path into two sections with a gap on either side of the label. IfFALSE
, the path is plotted as a whole. Alternatively, ifNA
, the path will be broken if the string has avjust
between 0 and 1, and not otherwise. The default for the label variant isFALSE
and for the text variant isNA
.upright
A
logical(1)
which ifTRUE
(default), inverts any text where the majority of letters would upside down along the path, to improve legibility. IfFALSE
, the path decides the orientation of text.halign
A
character(1)
describing how multi-line text should be justified. Can either be"center"
(default),"left"
or"right"
.offset
A
unit
object of length 1 to determine the offset of the text from the path. If this isNULL
(default), thevjust
parameter decides the offset. If notNULL
, theoffset
argument overrules thevjust
setting.parse
A
logical(1)
which ifTRUE
, will coerce the labels into expressions, allowing for plotmath syntax to be used.straight
A
logical(1)
which ifTRUE
, keeps the letters of a label on a straight baseline and ifFALSE
(default), lets individual letters follow the curve. This might be helpful for noisy paths.padding
A
unit
object of length 1 to determine the padding between the text and the path when thegap
parameter trims the path.text_smoothing
a
numeric(1)
value between 0 and 100 that smooths the text without affecting the line portion of the geom. The default value of0
means no smoothing is applied.rich
A
logical(1)
whether to interpret the text as html/markdown formatted rich text. Default:FALSE
. See also the rich text section of the details ingeom_textpath()
.remove_long
if TRUE, labels that are longer than their associated path will be removed.
- contour_var
Character string identifying the variable to contour by. Can be one of
"density"
,"ndensity"
, or"count"
. See the section on computed variables for details.- n
Number of grid points in each direction.
- h
Bandwidth (vector of length two). If
NULL
, estimated usingMASS::bandwidth.nrd()
.- adjust
A multiplicative bandwidth adjustment to be used if 'h' is 'NULL'. This makes it possible to adjust the bandwidth while still using the a bandwidth estimator. For example,
adjust = 1/2
means use half of the default bandwidth.- lineend
Line end style (round, butt, square).
- linejoin
Line join style (round, mitre, bevel).
- linemitre
Line mitre limit (number greater than 1).
- na.rm
If
FALSE
, the default, missing values are removed with a warning. IfTRUE
, missing values are silently removed.- show.legend
logical. Should this layer be included in the legends?
NA
, the default, includes if any aesthetics are mapped.FALSE
never includes, andTRUE
always includes. It can also be a named logical vector to finely select the aesthetics to display.- inherit.aes
If
FALSE
, overrides the default aesthetics, rather than combining with them. This is most useful for helper functions that define both data and aesthetics and shouldn't inherit behaviour from the default plot specification, e.g.borders()
.- label.padding
Amount of padding around label. Defaults to 0.25 lines.
- label.r
Radius of rounded corners. Defaults to 0.15 lines.
- arrow
Arrow specification, as created by
grid::arrow()
.
Aesthetics
geom_textdensity2d()
understands the following aesthetics (required aesthetics are in bold):
x
y
alpha
angle
colour
family
fontface
group
hjust
linecolour
lineheight
linetype
linewidth
size
spacing
textcolour
vjust
In addition to aforementioned aesthetics, geom_labeldensity2d()
also understands:
boxcolour
boxlinetype
boxlinewidth
fill
The spacing
aesthetic allows fine control of spacing
of text, which is called 'tracking' in typography.
The default is 0 and units are measured in 1/1000 em.
Numbers greater than zero increase the spacing,
whereas negative numbers decrease the spacing.
Learn more about setting these aesthetics
in vignette("ggplot2-specs")
.
See also
Other geom layers that place text on paths.
Examples
set.seed(1)
df <- data.frame(x = rnorm(100), y = rnorm(100))
ggplot(df, aes(x, y)) +
geom_textdensity2d() +
theme_classic()